1. 前言
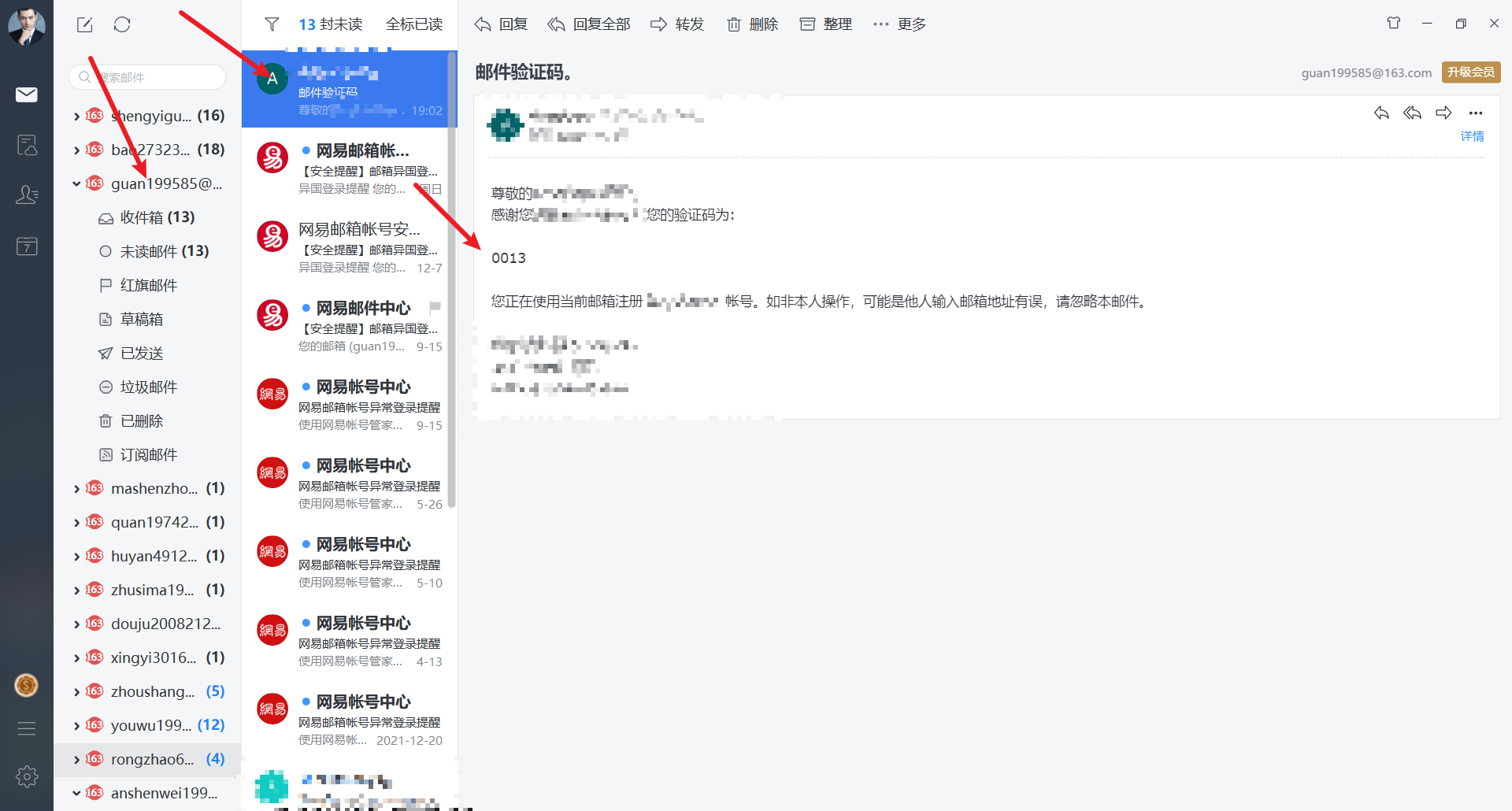
2. 效果
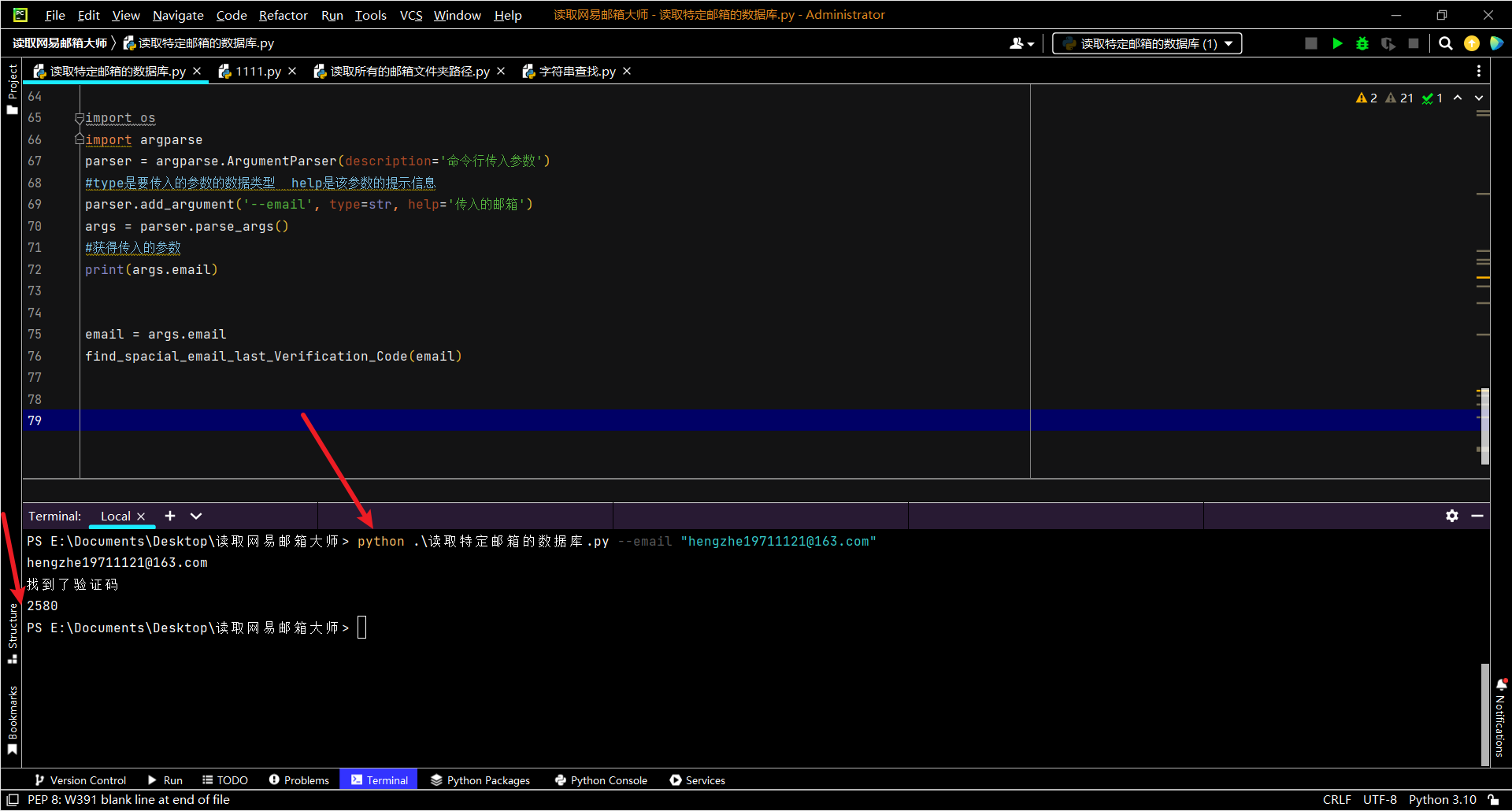
3. 探究过程
3.1. 找到本地存储的数据库
那么首先需要找到网易邮箱大师的数据存储位置
系统设置这里可以看到网易邮箱的数据存储位
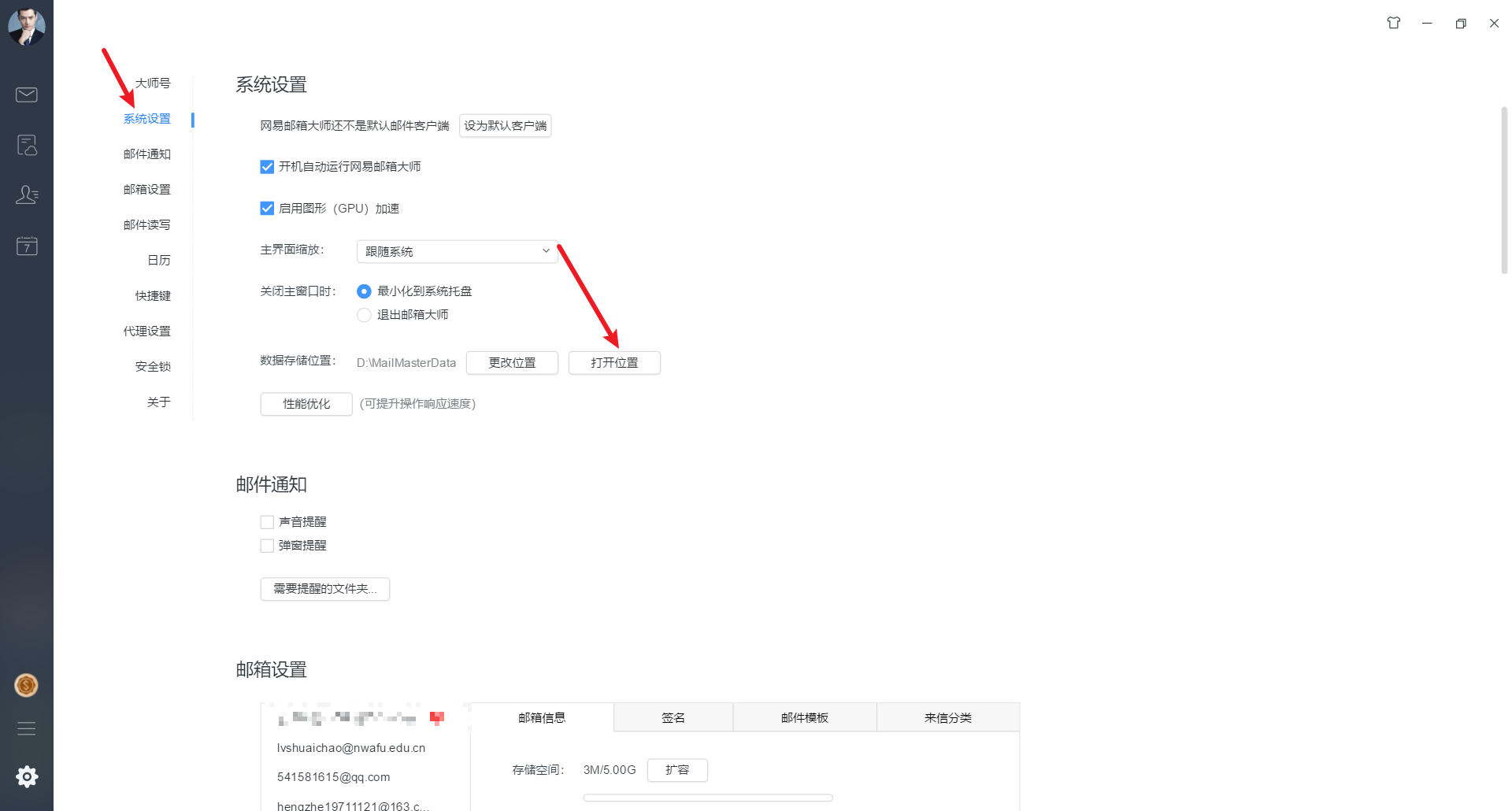
打开位置之后,是一系列以邮箱名命名的文件夹
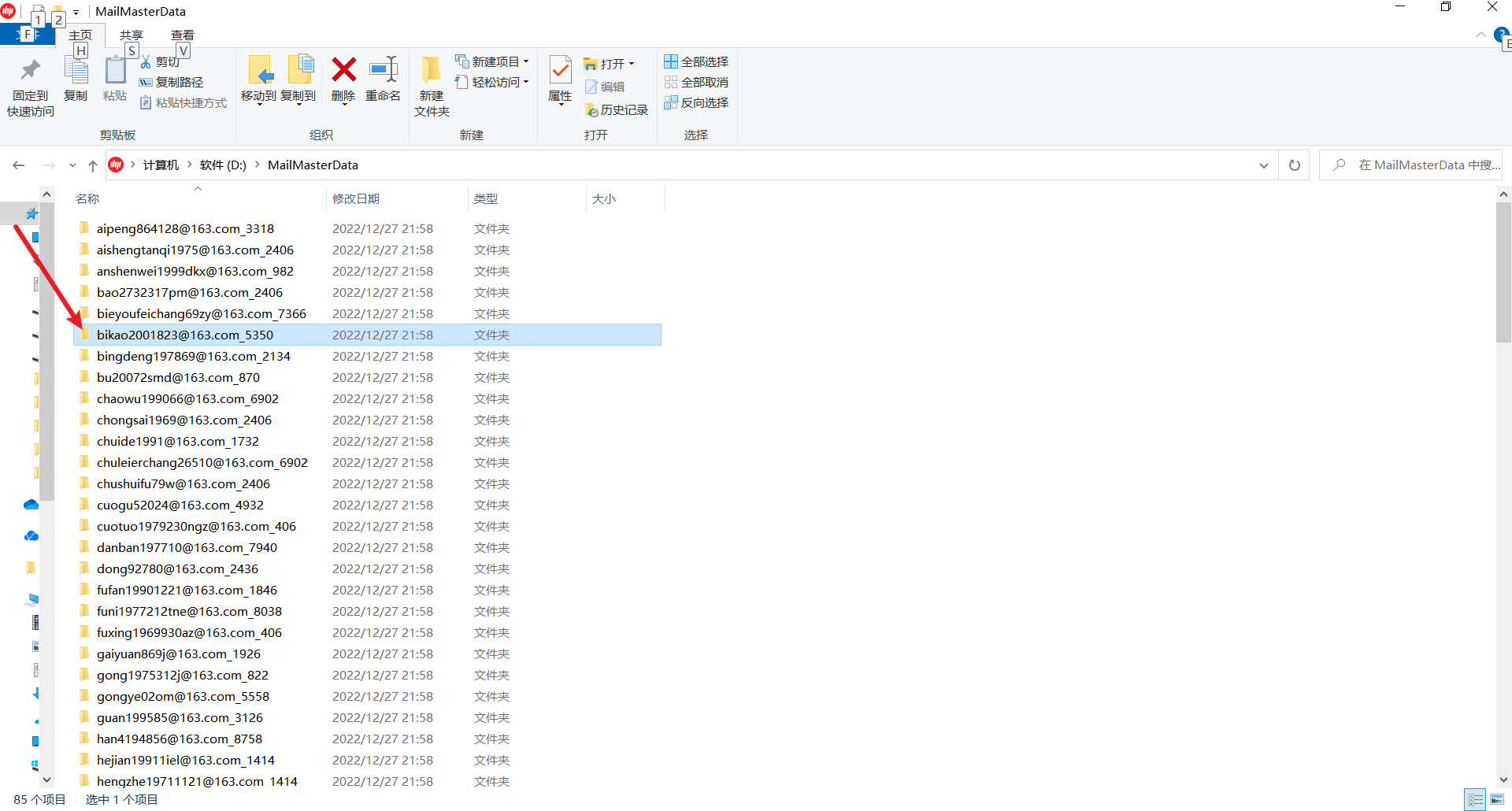
但是这里面的数据似乎是加密的数据库文件
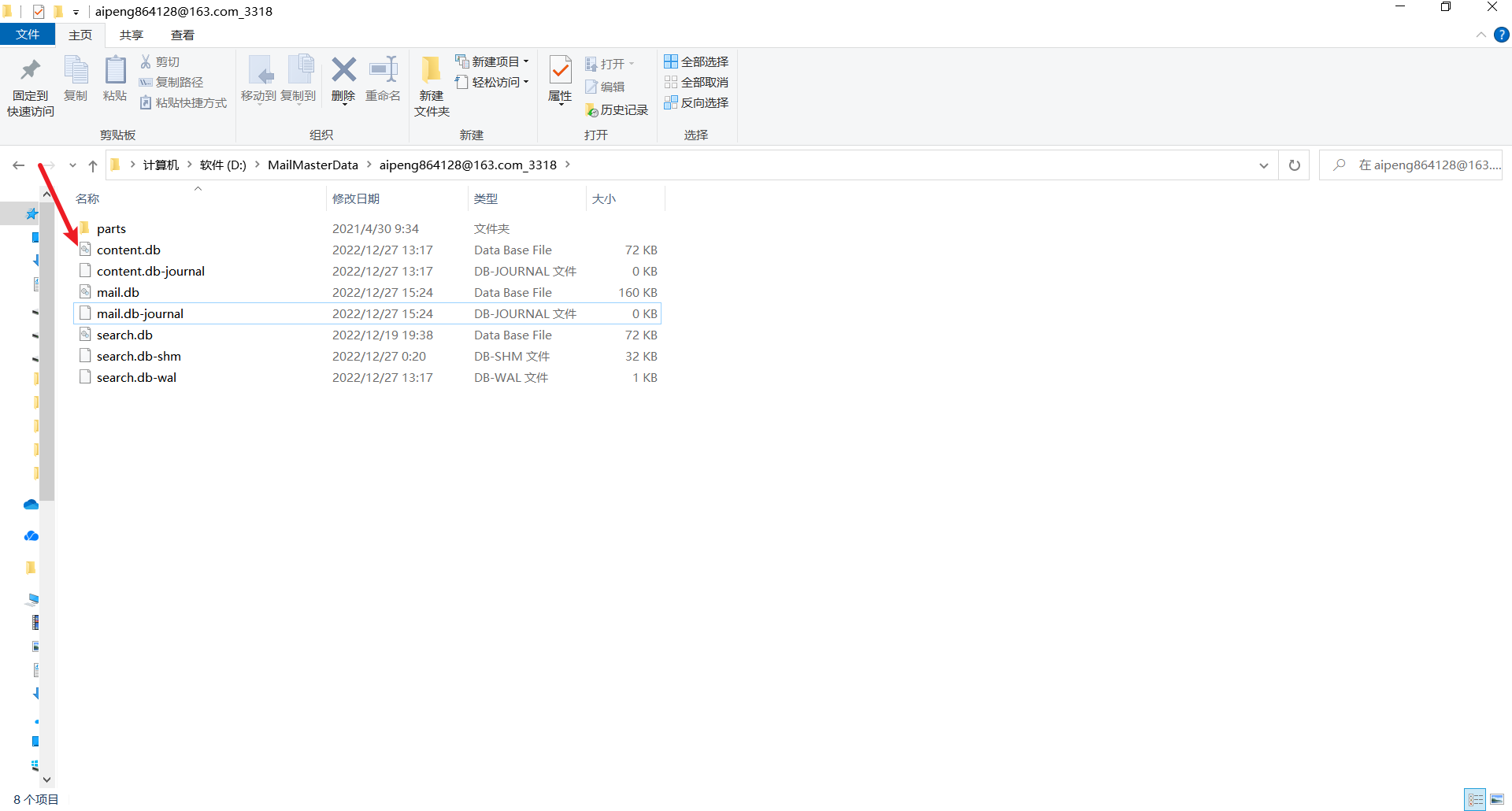
自己使用Navicat for sqlite打开发现也看不到里面的具体数据
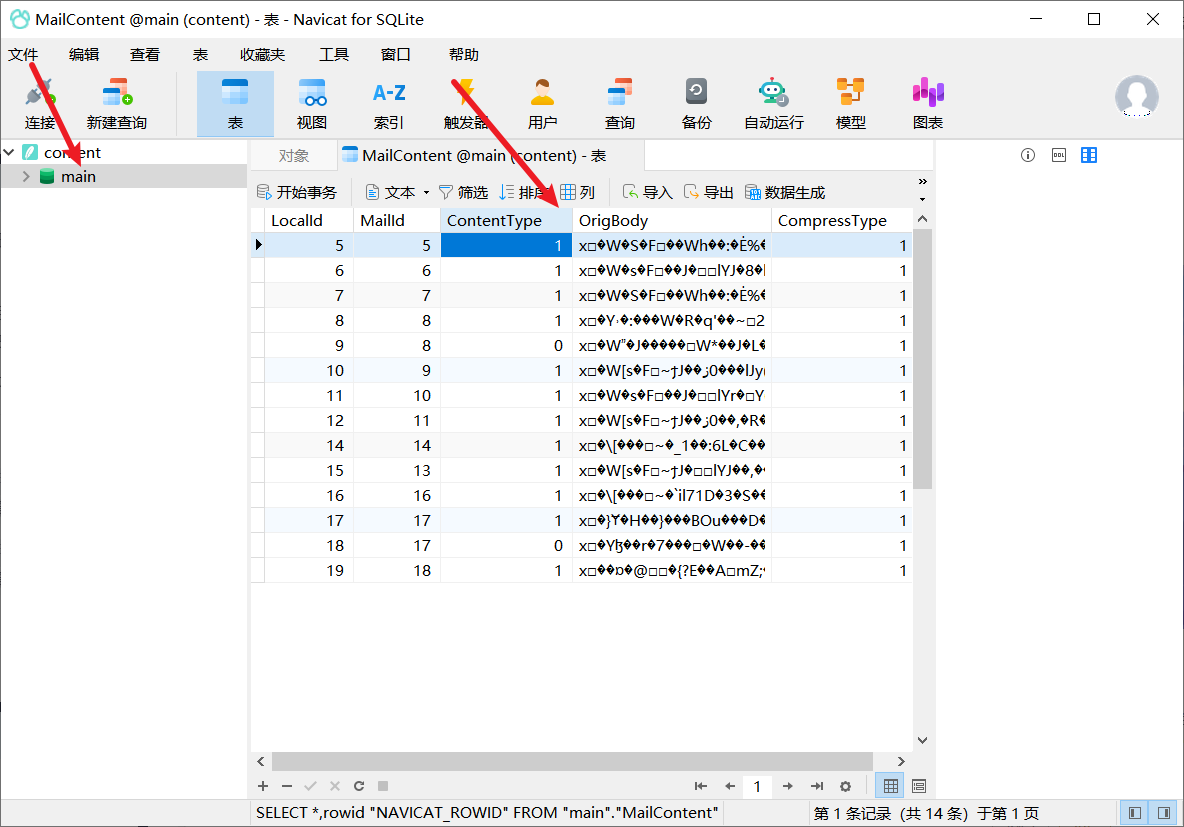
自己又换了一个db文件进行读取发现里面的数据似乎也并未完全加密,依稀可以看到一些邮件内容之前之所以显示是乱码,可能是转码的问题
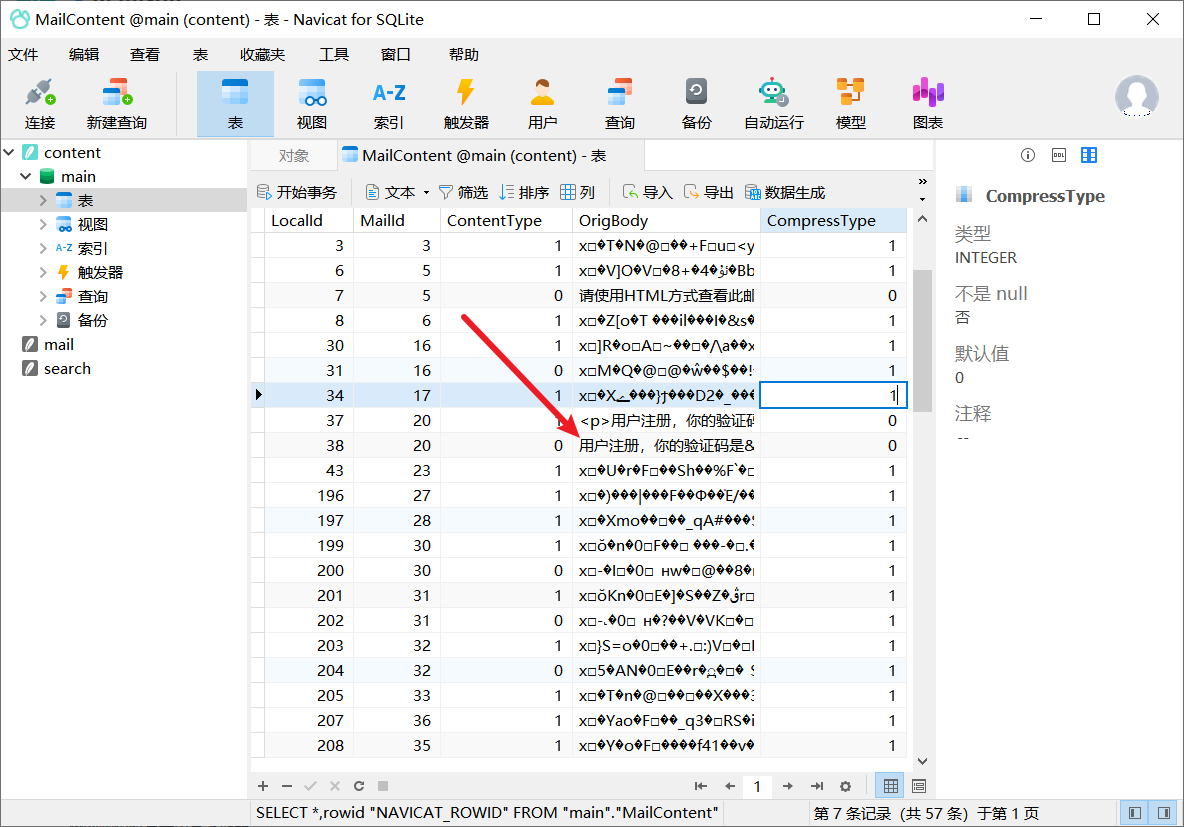
自己又查看了一下另外两个mail和search ,没想到邮件内容直接是在search/search_content中明文存储,看来今天的探索应该可以结束了
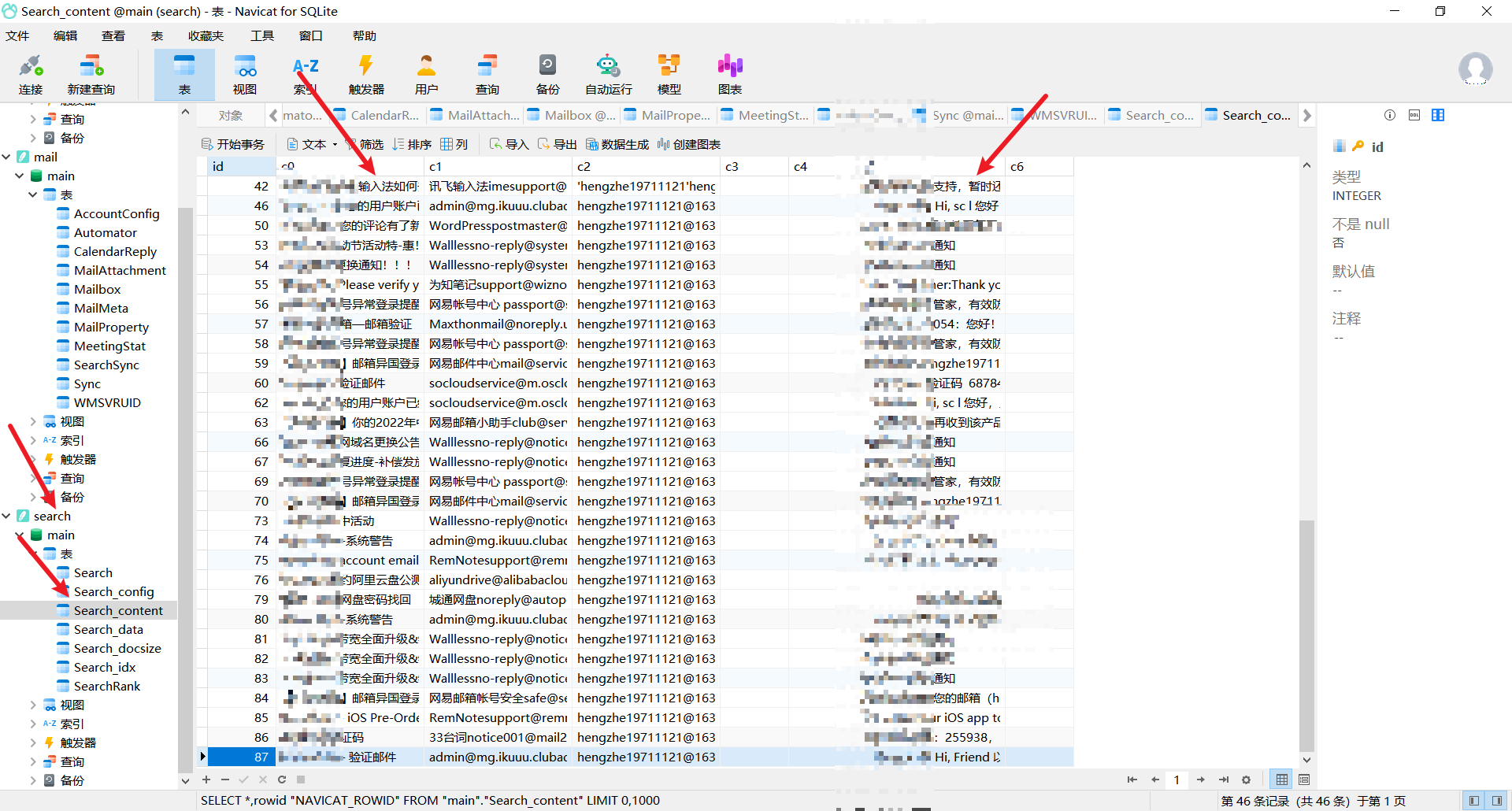
我们来新发一份邮件,看能否实时更新
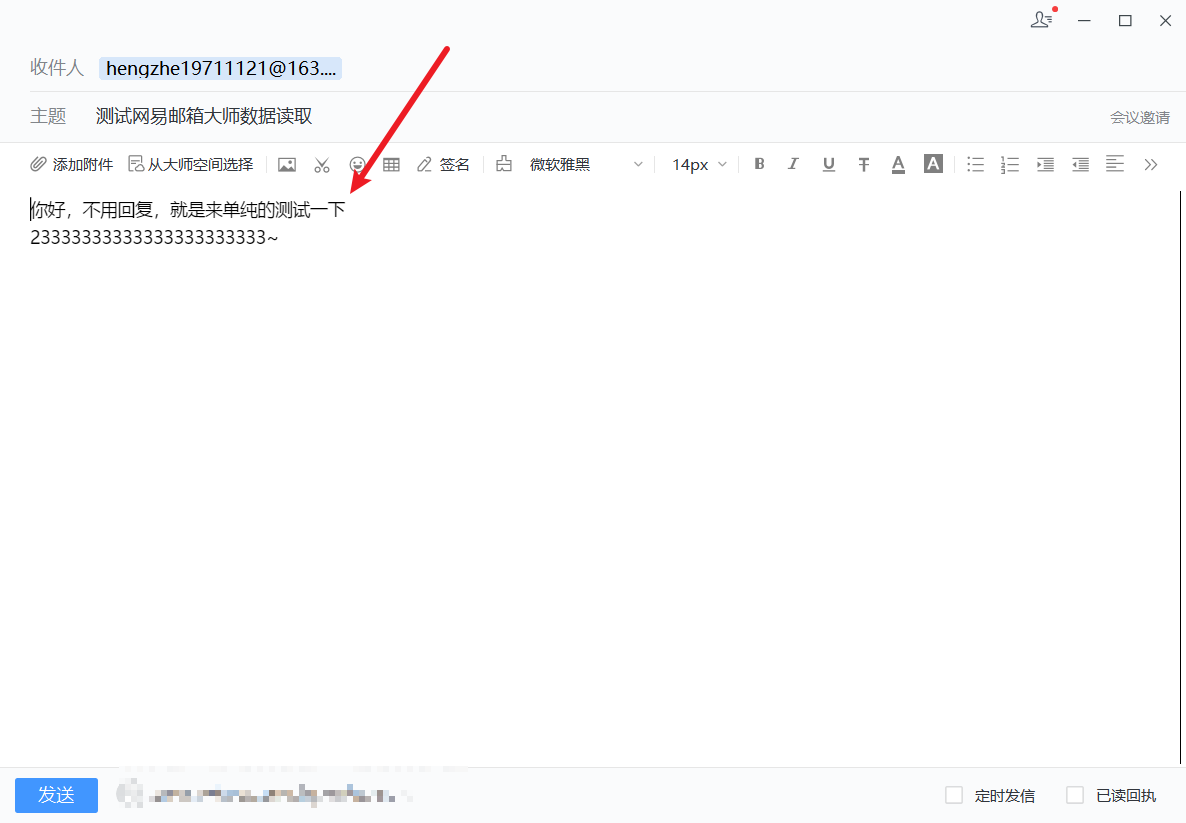
可能是自己添加的邮箱数量太多了,这里一直处于发送过程中
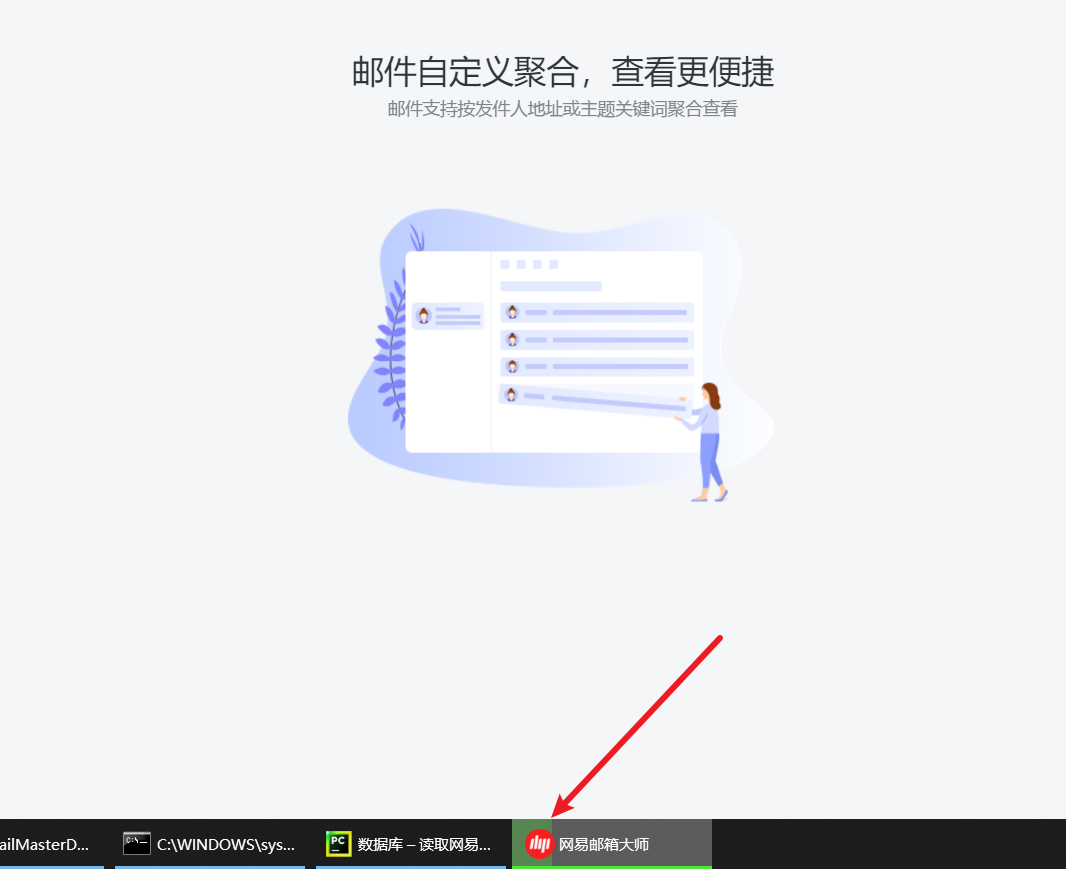
重启了一下网易邮箱客户端之后正常了
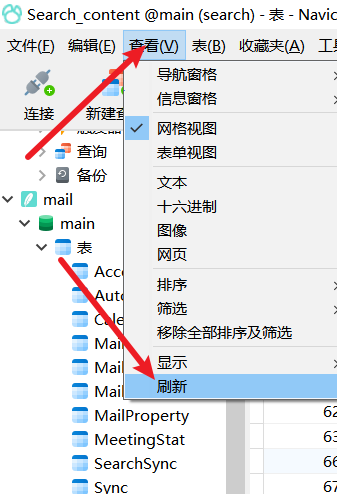
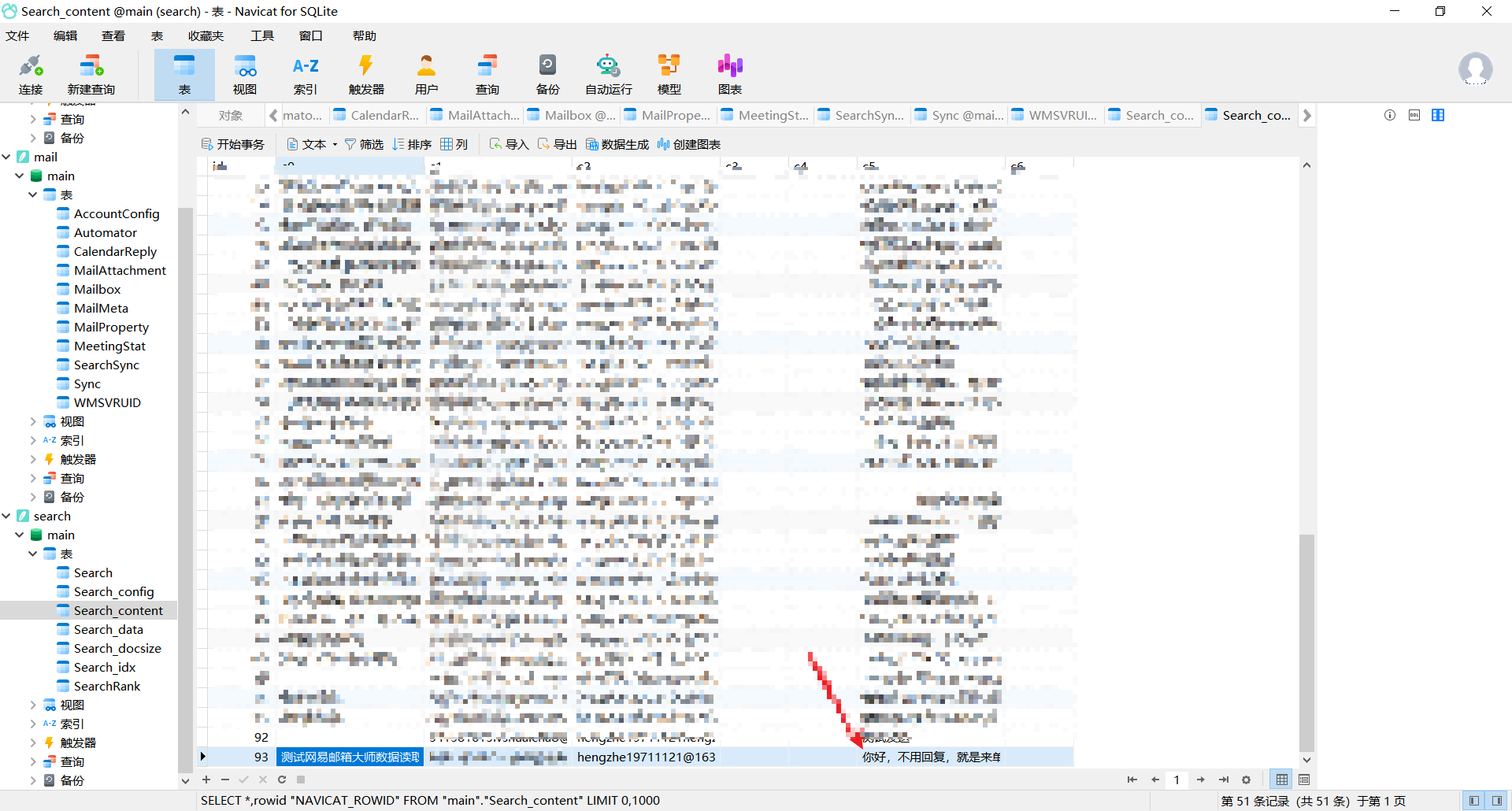
到这里证明我们的这个原理是行得通的了。那么下面就用程序来实现这个过程
3.2. 使用Python读取数据库
3.2.1. 代码
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('D:\MailMasterData\[email protected]_1414\search.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'select * from Search_content'
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
# print(person_all)
# 遍历
for p in person_all:
print(p)
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
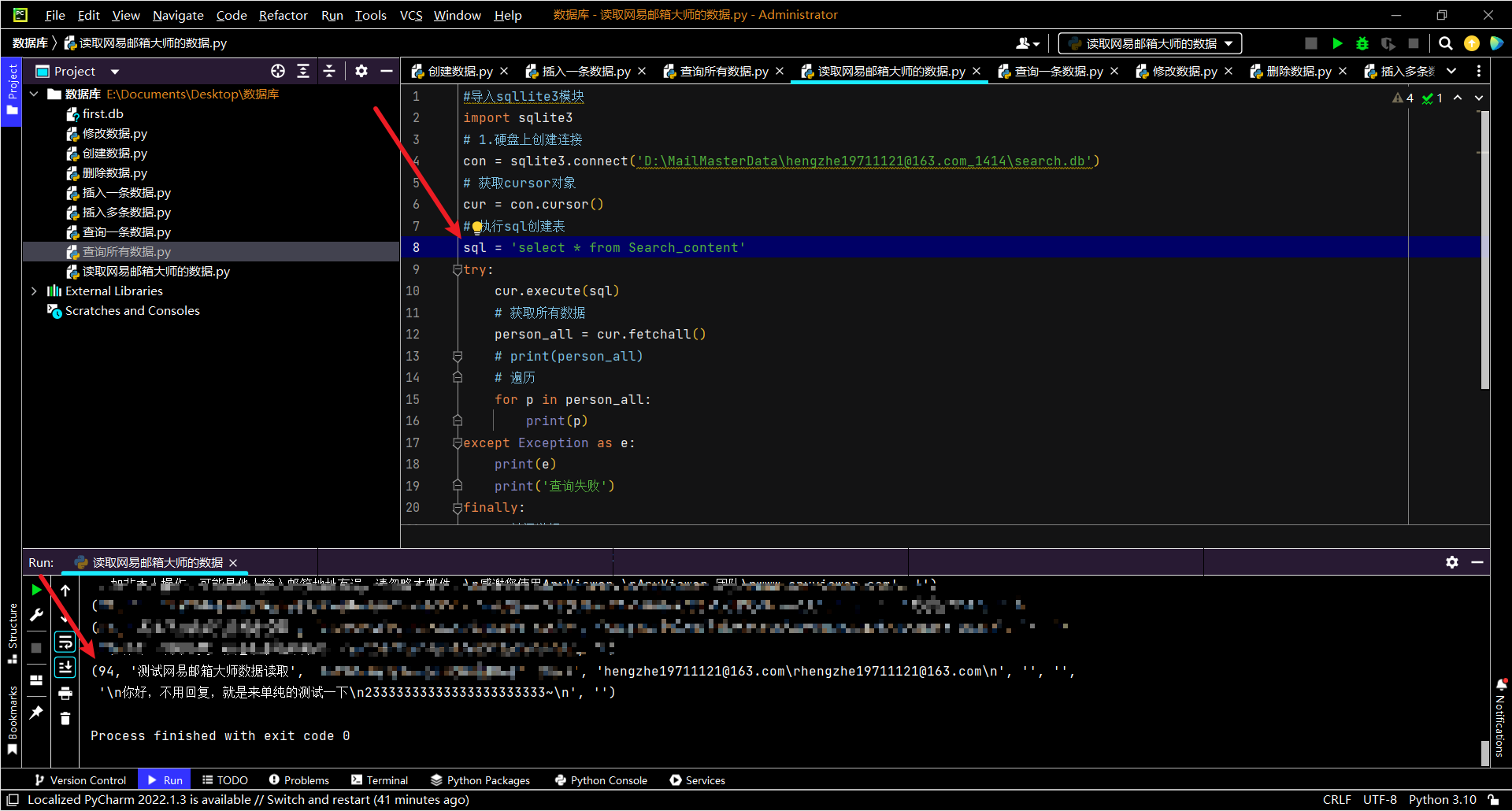
4. 探究结果
4.1. 函数
4.1.1. 找到特定邮~箱的最新一条邮件
def sqlite3_get_last_data(db_path,sql): 找到特定邮箱最后一次的数据
# 导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect(db_path)
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
last_data = person_all[-1]
# print(last_data)
# print("type(last_data):", type(last_data))
# print("last_data:", )
last_text = last_data[6]
return last_text
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
email_path = '[email protected]'
db_path = 'D:\MailMasterData\{}_1414\search.db'.format(email_path)
sql = 'select * from Search_content'
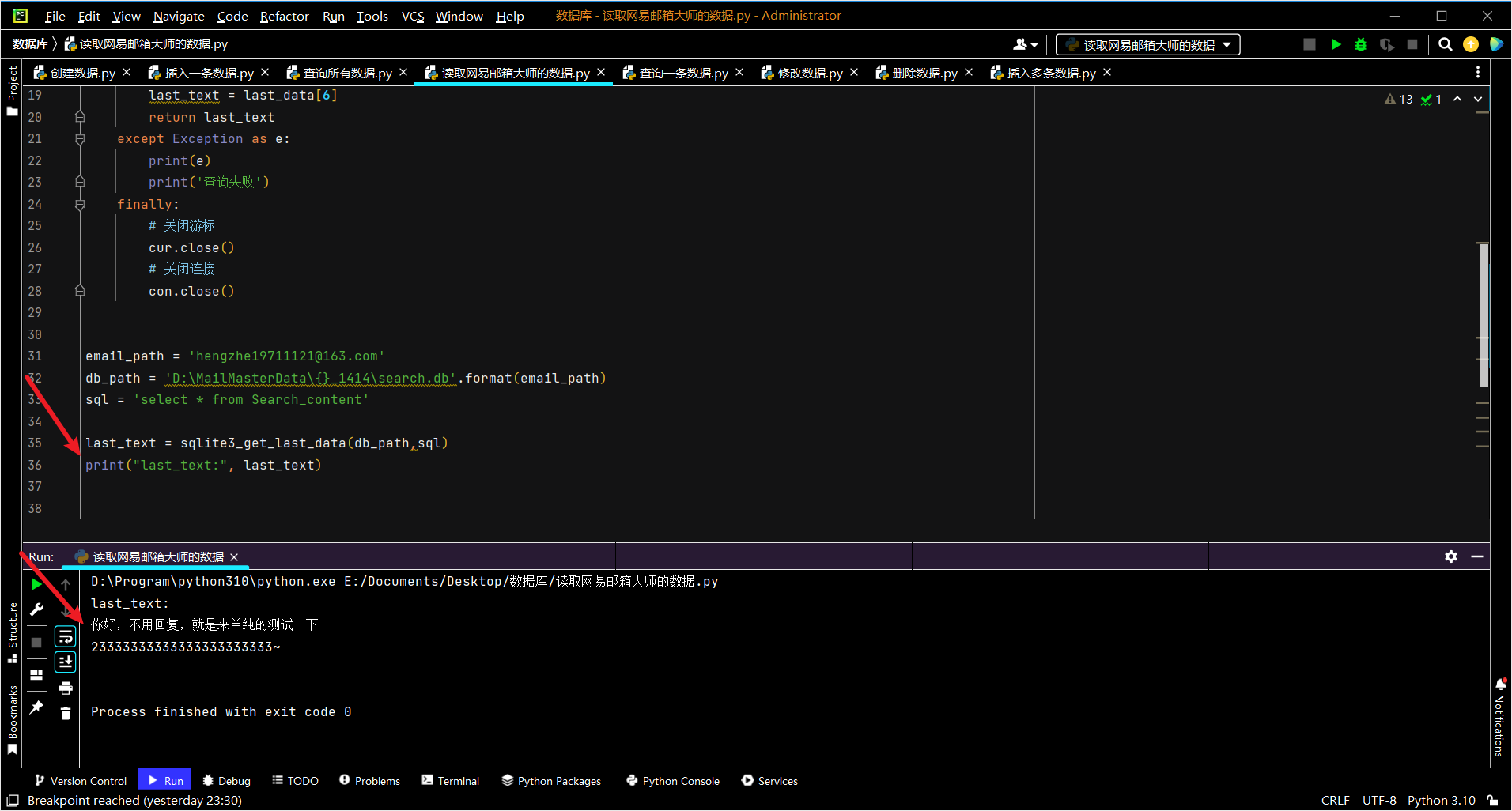
4.1.2. 找到特定邮箱的最新一次验证码
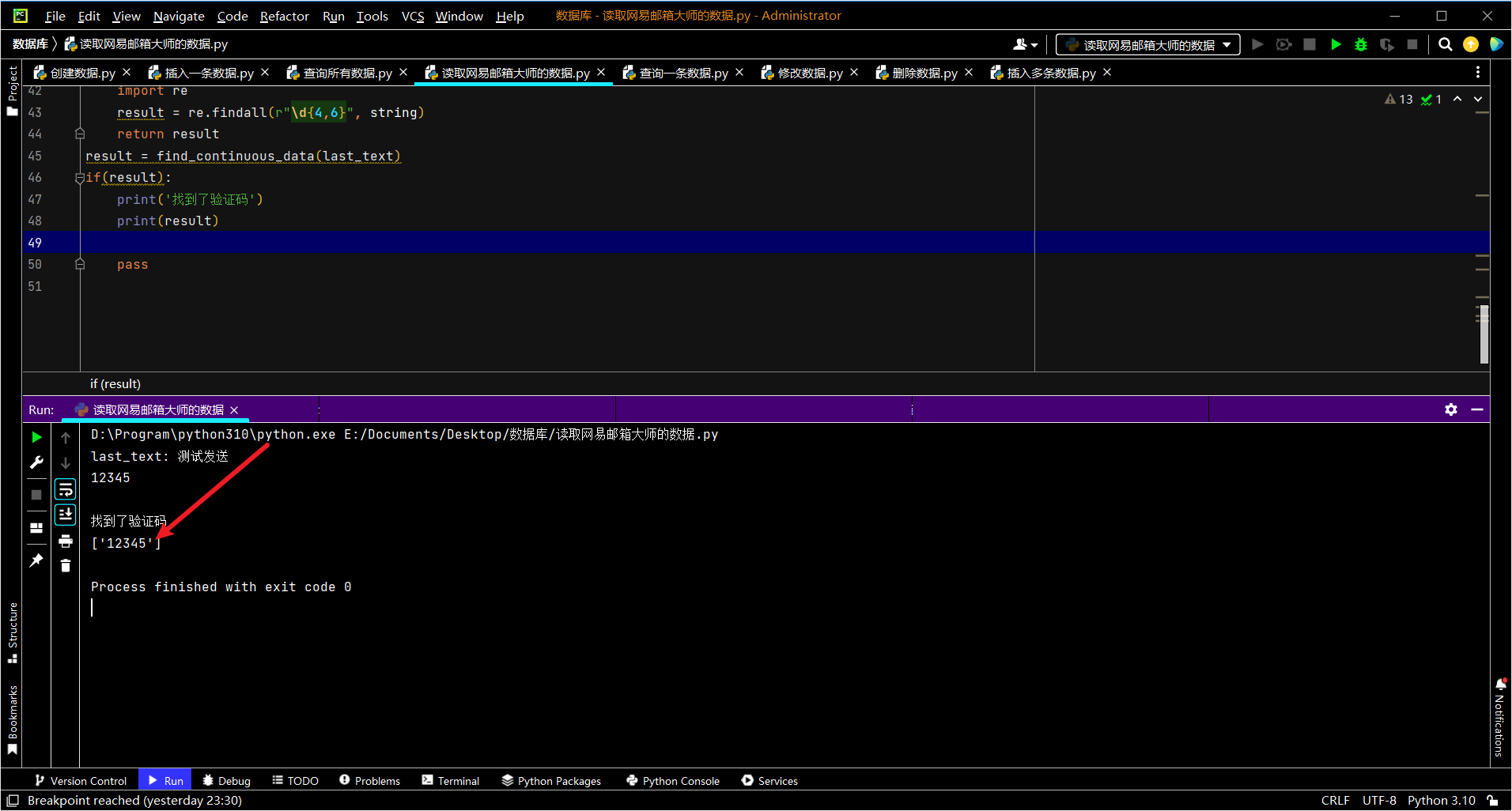
代码
def find_spacial_email_last_Verification_Code(email): #找到特定邮箱最后一次的验证码
def sqlite3_get_last_data(db_path, sql):
# 导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect(db_path)
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
last_data = person_all[-1]
# print(last_data)
# print("type(last_data):", type(last_data))
# print("last_data:", )
last_text = last_data[6]
return last_text
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
def find_continuous_data(string): # 查找字符串中连续的数字
import re
result = re.findall(r"\d{4,6}", string)
return result
def find_str_in_list_full_string(list_str, find_str): # 查找字符串列表中包含某一个字符的完整字符
for index, str in enumerate(list_str):
if find_str in str:
break
return str
import os
mailbox_rootpath = 'D:\MailMasterData'
email_name_list = os.listdir(mailbox_rootpath)
email_dir_name = find_str_in_list_full_string(email_name_list, email)
db_path = '{}\{}\search.db'.format(mailbox_rootpath,email_dir_name)
sql = 'select * from Search_content'
last_text = sqlite3_get_last_data(db_path, sql)
print("last_text:", last_text)
result = find_continuous_data(last_text)
if (len(result) == 1):
print('找到了验证码')
print(result[0])
pass
else:
from winsound import Beep
Beep(600, 1000)
email = '[email protected]'
find_spacial_email_last_Verification_Code(email)
4.1.3. 通过命令行调用Python代码找到特定邮箱的最新的验证码
代码
def find_spacial_email_last_Verification_Code(email): #找到特定邮箱最后一次的验证码
def sqlite3_get_last_data(db_path, sql): #找到特定邮箱最后一次的数据
# 导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect(db_path)
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
last_data = person_all[-1]
# print(last_data)
# print("type(last_data):", type(last_data))
# print("last_data:", )
last_text = last_data[6]
return last_text
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
def find_continuous_data(string): # 查找字符串中连续的数字
import re
result = re.findall(r"\d{4,6}", string)
return result
def find_str_in_list_full_string(list_str, find_str): # 查找字符串列表中包含某一个字符的完整字符
for index, str in enumerate(list_str):
if find_str in str:
break
return str
import os
mailbox_rootpath = 'D:\MailMasterData'
email_name_list = os.listdir(mailbox_rootpath)
email_dir_name = find_str_in_list_full_string(email_name_list, email)
db_path = '{}\{}\search.db'.format(mailbox_rootpath,email_dir_name)
sql = 'select * from Search_content'
last_text = sqlite3_get_last_data(db_path, sql)
# print("last_text:", last_text)
result = find_continuous_data(last_text)
if (len(result) == 1):
print('找到了验证码')
print(result[0])
pass
else:
from winsound import Beep
Beep(600, 1000)
import os
import argparse
parser = argparse.ArgumentParser(description='命令行传入参数')
#type是要传入的参数的数据类型 help是该参数的提示信息
parser.add_argument('--email', type=str, help='传入的邮箱')
args = parser.parse_args()
#获得传入的参数
print(args.email)
email = args.email
find_spacial_email_last_Verification_Code(email)
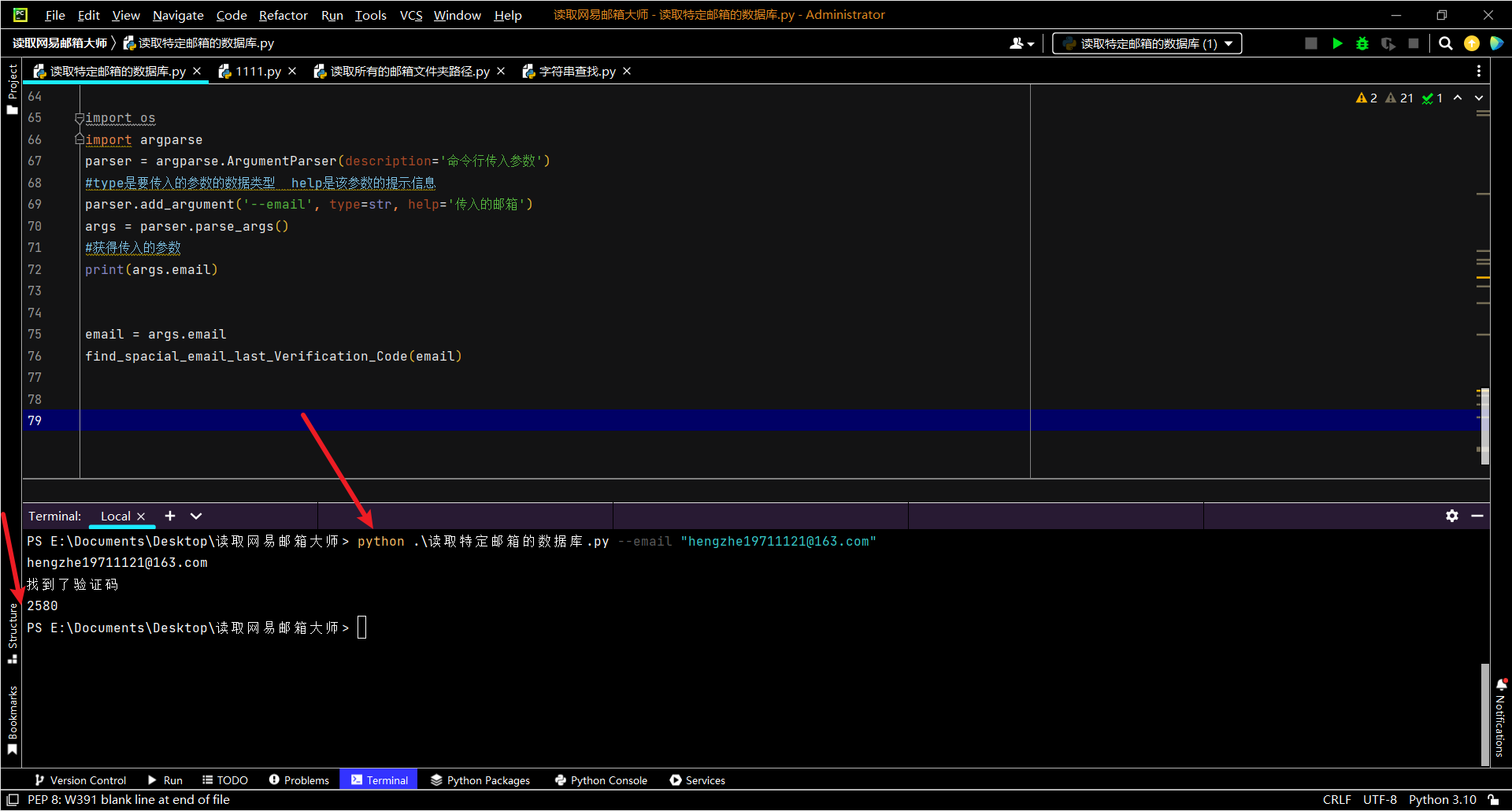