1. 简介
1.1. 使用
1.1.1. 创建
代码
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'create table t_person(pno INTEGER PRIMARY KEY AUTOINCREMENT ,pname varchar(30) NOT NULL ,age INTEGER)'
try:
cur.execute(sql)
except Exception as e:
print(e)
print('创建表失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
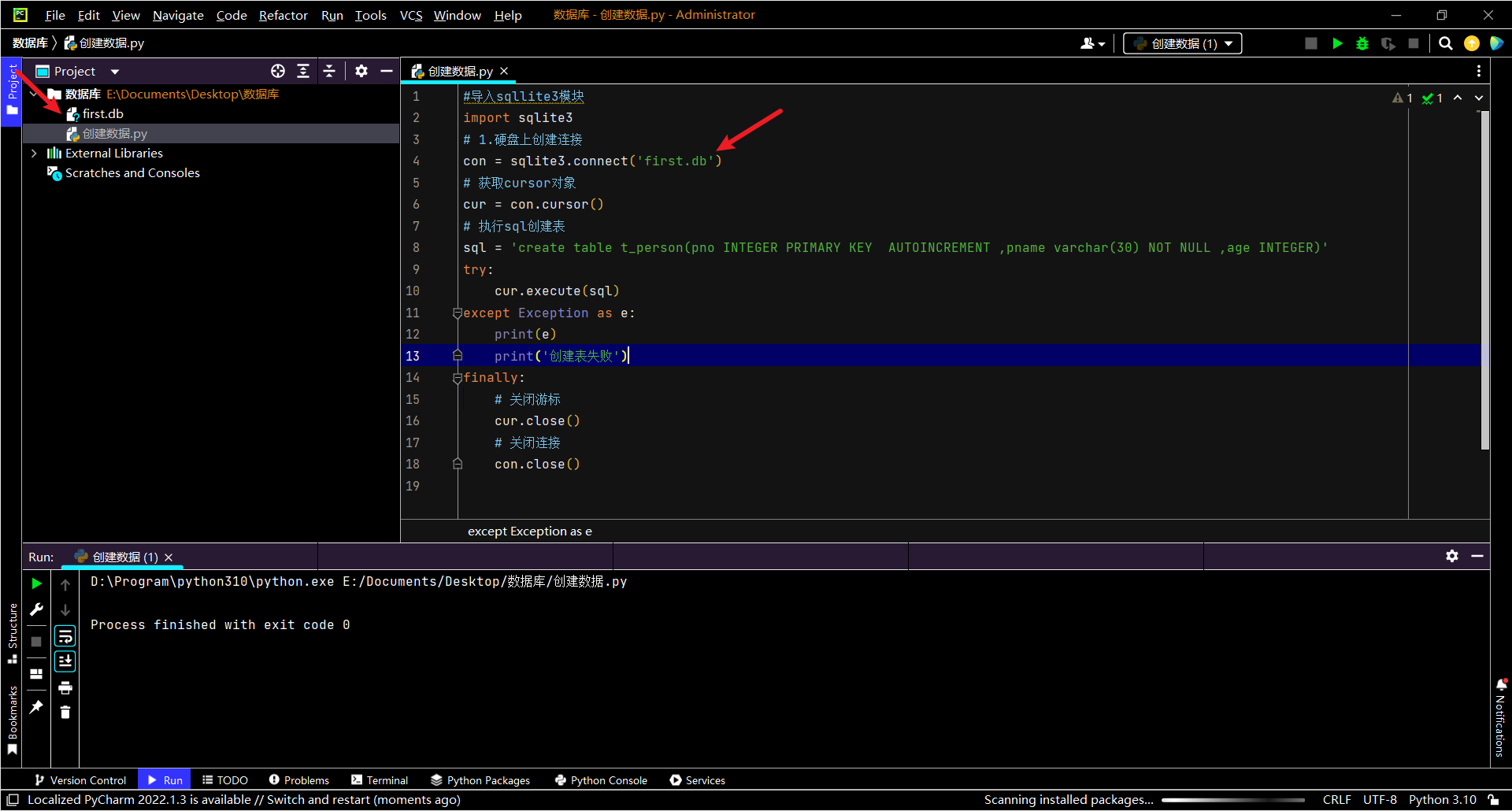
1.1.2. 插入
1.1.2.1. 插入一条数据
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'insert into t_person(pname,age) values(?,?)'
try:
cur.execute(sql,('张三',23))
#提交事务
con.commit()
print('插入成功')
except Exception as e:
print(e)
print('插入失败')
con.rollback()
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
1.1.3. 查询
1.1.3.1. 查询所有数据
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'select * from t_person'
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
# print(person_all)
# 遍历
for p in person_all:
print(p)
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
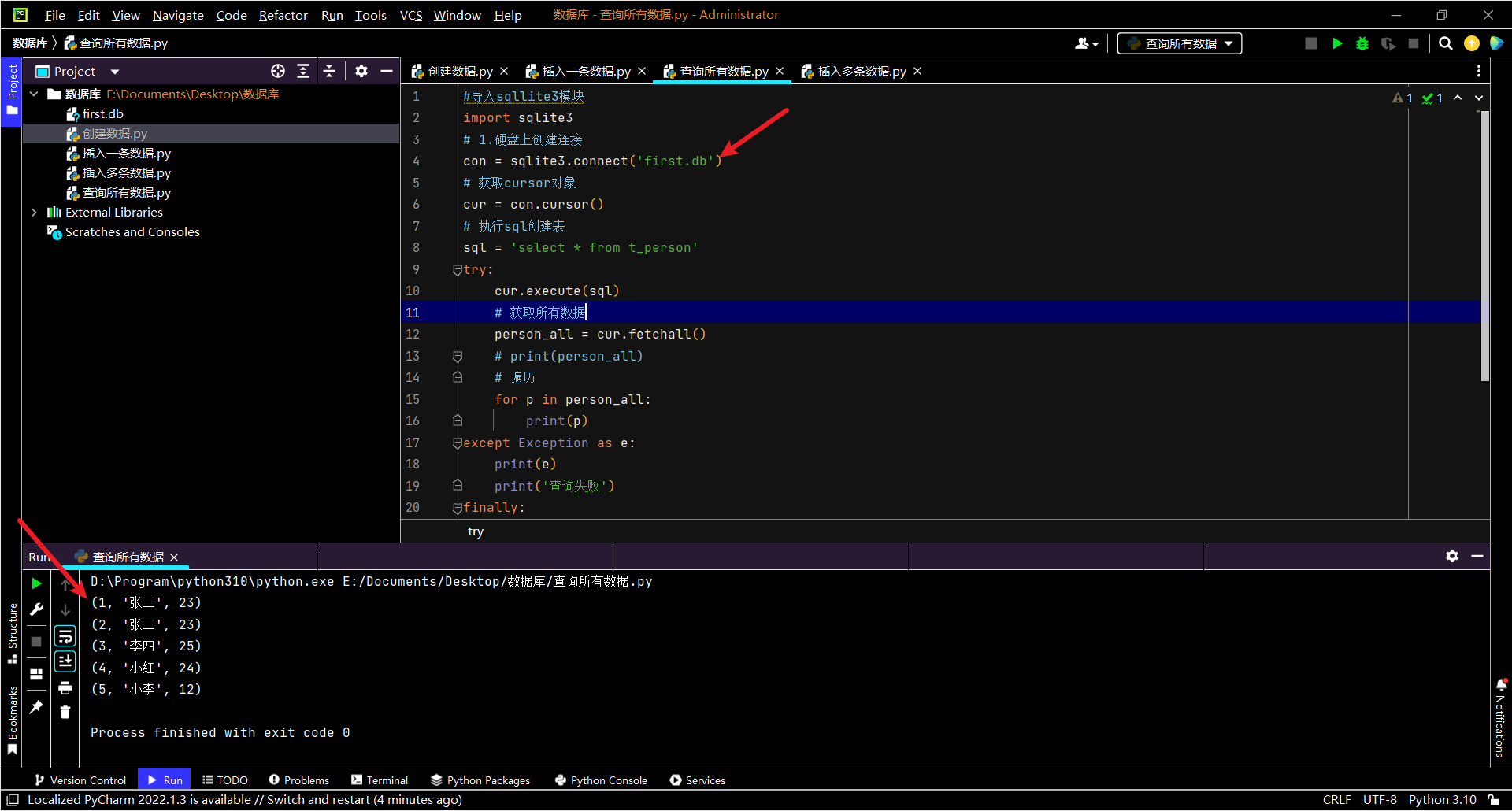
查询一条数据
fetchone()查询一条数据
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'select * from t_person'
try:
cur.execute(sql)
# 获取一条数据
person = cur.fetchone()
print(person)
person = cur.fetchone()
print(person)
person = cur.fetchone()
print(person)
person = cur.fetchone()
print(person)
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
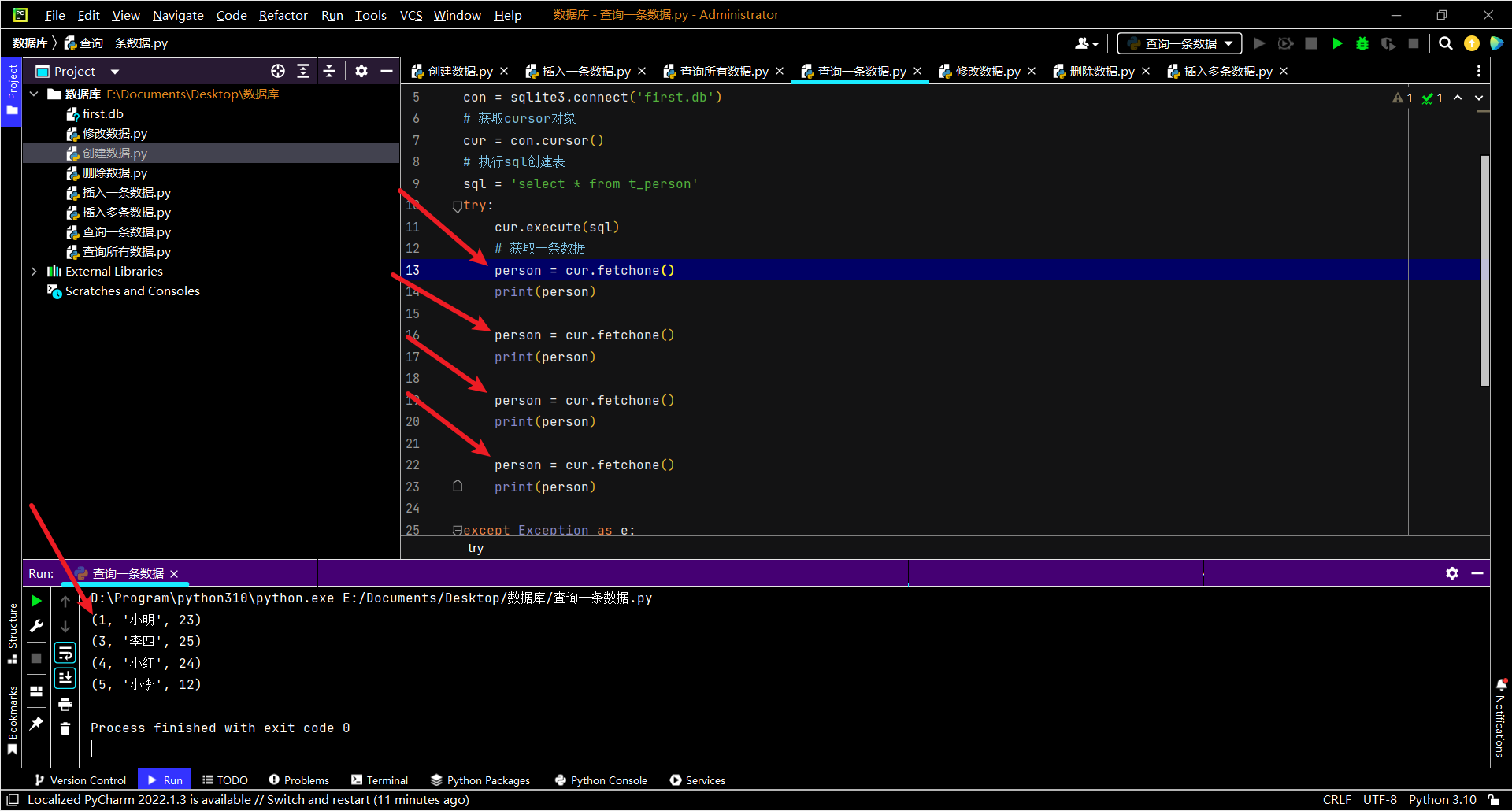
1.1.3.2. 读取特定位置的数据
获得最新一条数据
def sqlite3_get_last_data(db_path,sql):
# 导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect(db_path)
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
last_data = person_all[-1]
# print(last_data)
# print("type(last_data):", type(last_data))
# print("last_data:", )
last_text = last_data[6]
return last_text
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
db_path = 'D:\MailMasterData\[email protected]_1414\search.db'
sql = 'select * from Search_content'
last_text = sqlite3_get_last_data(db_path,sql)
print("last_text:", last_text)
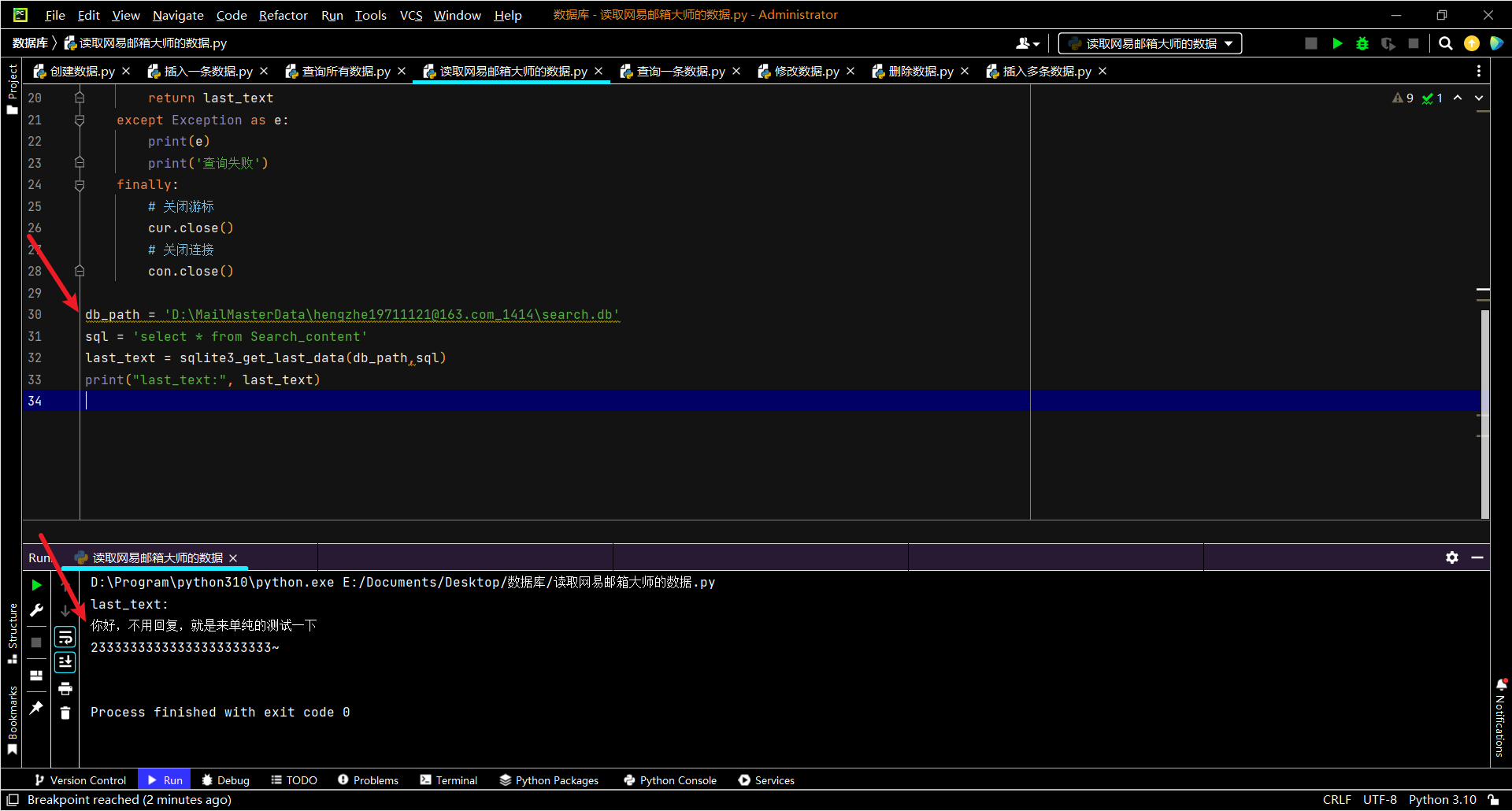
1.1.4. 修改数据
代码
#导入sqllite3模块
import sqlite3
#1.硬盘上创建连接
con=sqlite3.connect('first.db')
#获取cursor对象
cur=con.cursor()
try:
#执行sql创建表
update_sql = 'update t_person set pname=? where pno=?'
cur.execute(update_sql, ('小明', 1))
#提交事务
con.commit()
print('修改成功')
except Exception as e:
print(e)
print('修改失败')
con.rollback()
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
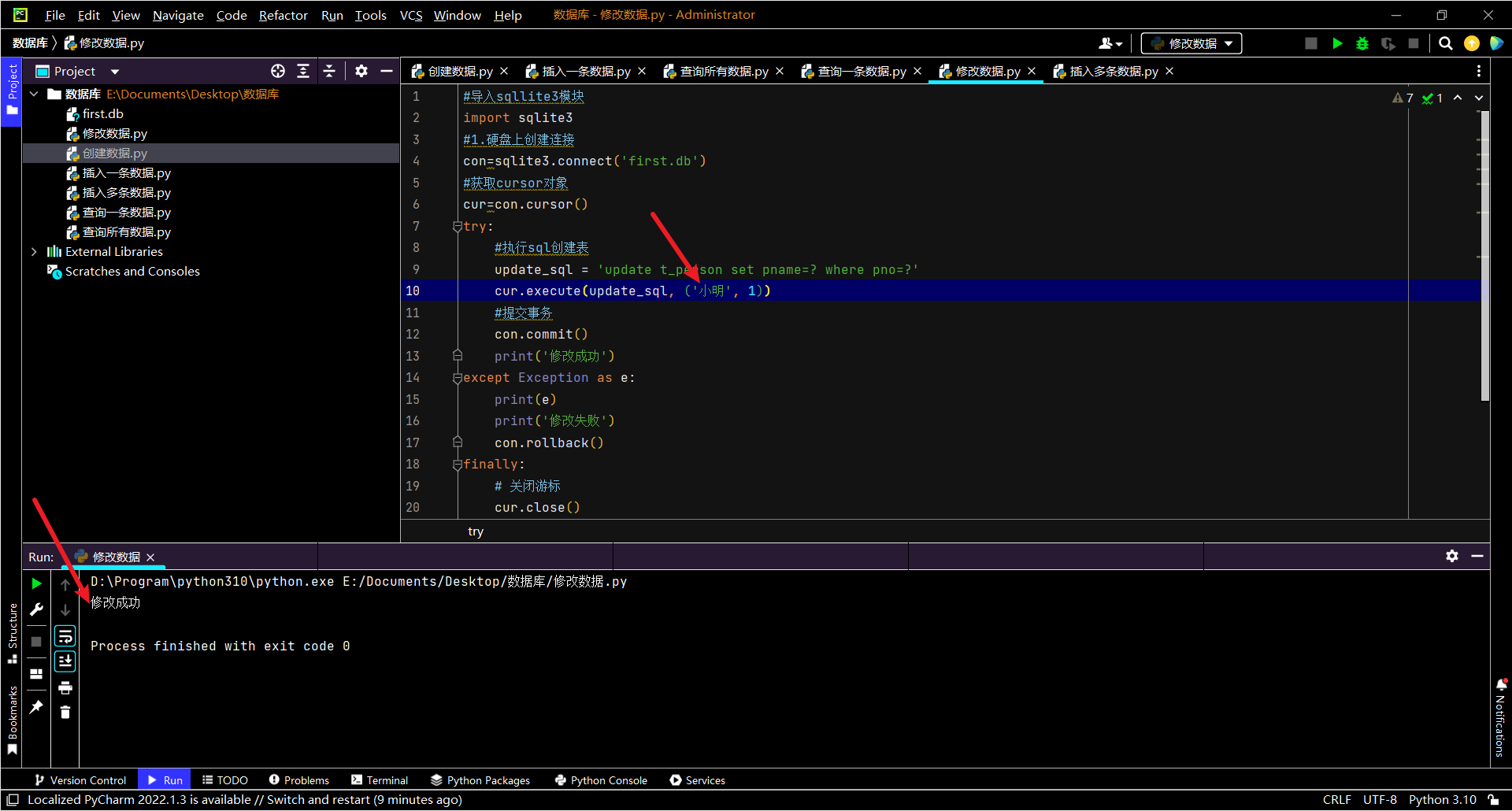
1.1.5. 删除数据
代码
#导入sqllite3模块
import sqlite3
#1.硬盘上创建连接
con=sqlite3.connect('first.db')
#获取cursor对象
cur=con.cursor()
#执行sql创建表
delete_sql = 'delete from t_person where pno=?'
try:
cur.execute(delete_sql, (2,))
#提交事务
con.commit()
print('删除成功')
except Exception as e:
print(e)
print('删除失败')
con.rollback()
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
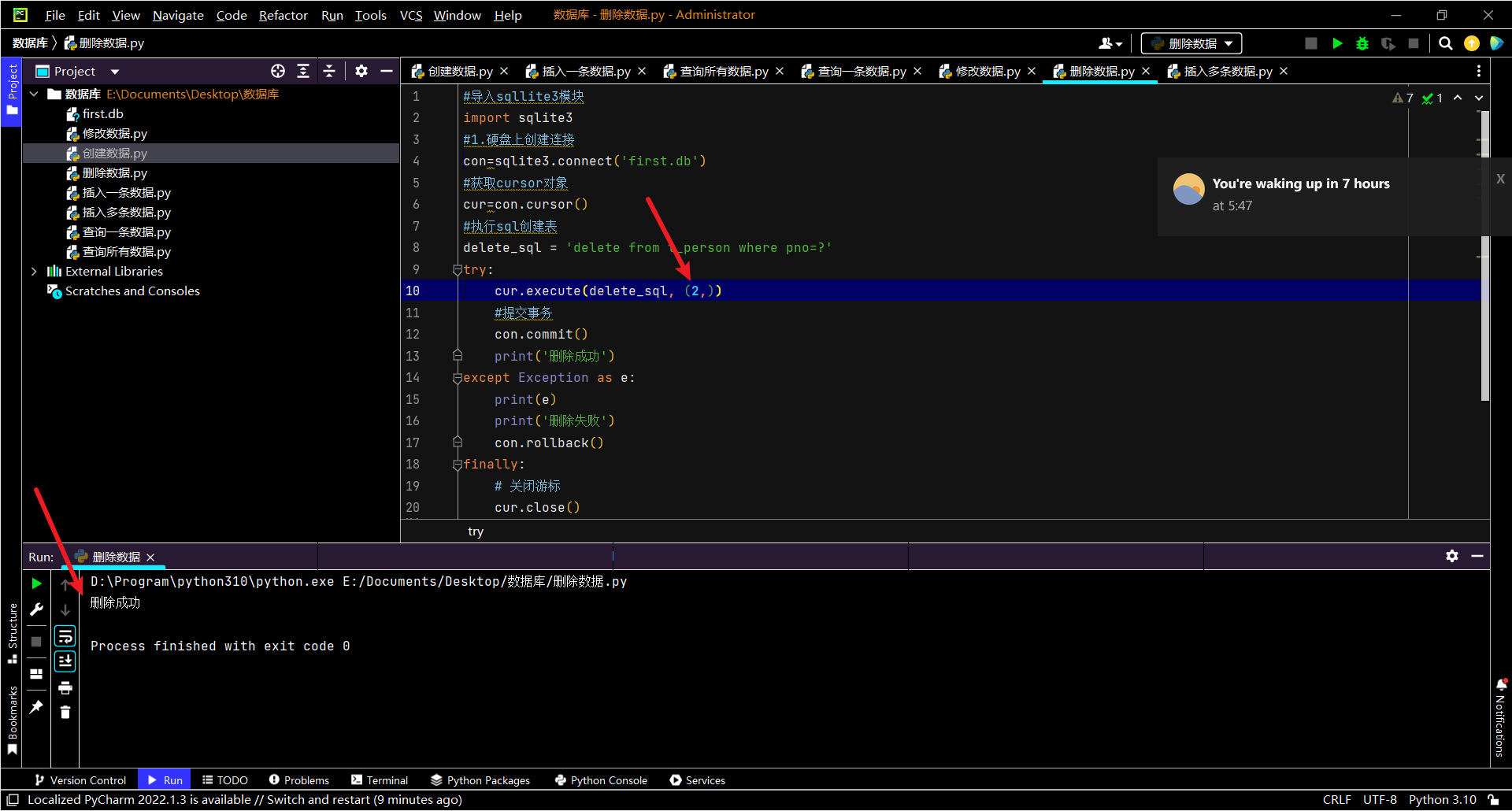